RunTS
mth5.timeseries.RunTS
is a container to hold multiple synchronous channels of the same sampling rate. The data is contained in an xarray.DataSet
which is a collection of ChannelTS.to_xarray()
objects.
[1]:
%matplotlib inline
import numpy as np
from mth5.timeseries import ChannelTS, RunTS
from mt_metadata.timeseries import Electric, Magnetic, Auxiliary, Run, Station
2021-11-17 20:09:31,114 [line 135] mth5.setup_logger - INFO: Logging file can be found C:\Users\jpeacock\Documents\GitHub\mth5\logs\mth5_debug.log
Create a Run
We will create a common run that has all 5 channels of an MT measurement (Hx, Hy, Hz, Ex, Ey) plus an auxiliary channel. We will make individual channels first and then add them into a RunTS
object.
[2]:
channel_list = []
common_start = "2020-01-01T00:00:00"
sample_rate = 1.0
n_samples = 4096
t = np.arange(n_samples)
data = np.sum([np.cos(2*np.pi*w*t + phi) for w, phi in zip(np.logspace(-3, 3, 20), np.random.rand(20))], axis=0)
station_metadata = Station(id="mt001")
run_metadata = Run(id="001")
Create magnetic
channels
[3]:
for component in ["hx", "hy", "hz"]:
h_metadata = Magnetic(component=component)
h_metadata.time_period.start = common_start
h_metadata.sample_rate = sample_rate
h_channel = ChannelTS(
channel_type="magnetic",
data=data,
channel_metadata=h_metadata,
run_metadata=run_metadata,
station_metadata=station_metadata)
channel_list.append(h_channel)
Create electric
channels
[4]:
for component in ["ex", "ey"]:
e_metadata = Electric(component=component)
e_metadata.time_period.start = common_start
e_metadata.sample_rate = sample_rate
e_channel = ChannelTS(
channel_type="electric",
data=data,
channel_metadata=e_metadata,
run_metadata=run_metadata,
station_metadata=station_metadata)
channel_list.append(e_channel)
Create auxiliary
channel
[5]:
aux_metadata = Auxiliary(component="temperature")
aux_metadata.time_period.start = common_start
aux_metadata.sample_rate = sample_rate
aux_channel = ChannelTS(
channel_type="auxiliary",
data=np.random.rand(n_samples) * 30,
channel_metadata=aux_metadata,
run_metadata=run_metadata,
station_metadata=station_metadata)
channel_list.append(aux_channel)
Create RunTS
object
Now that we have made individual channels we can make a RunTS
object by inputing a list of ChannelTS
objects.
Note: This can also be a list of xarray.DataArray
objects formated like a channel.
[6]:
run = RunTS(channel_list)
[7]:
run
[7]:
RunTS Summary:
Station: mt001
Run: 001
Start: 2020-01-01T00:00:00+00:00
End: 2020-01-01T01:08:15+00:00
Sample Rate: 1.0
Components: ['hx', 'hy', 'hz', 'ex', 'ey', 'temperature']
Plot Run
Again this is a hack at the moment, we are working on a better visualization, but this works for now.
[8]:
run.plot()
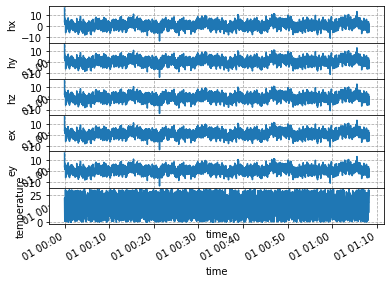